Sending E-mails with JavaMail on Glassfish V3
One of the main advantages of using an application server like Glassfish is to keep your application free of complex code, such as 1) manual control of database transactions; 2) database access configuration; 3) security authentication and authorization; 4) sending and receiving e-mail messages, among many other complexities that are non-functional requirements, consuming the time we would be spending on functional requirements.
In my opinion, an important differential of Glassfish V3 is its very rich and complete administration console. It is easy to use and to learn, which is, in my opinion, one of the most important competitive advantages, since it contributes to reduce the maintenance cost, a constant headache for system administrators. We are going to use the administrative console in order to configure a JavaMail resource for applications that aim to send emails**.
Follow the steps below:
- enter in the administrative console (http://[server-name]:4848/)
- go to Resources / JavaMail Sessions
- create a new JavaMail session and set the following mandatory properties:
- JNDI Name: mail/[email-account-name]
- Mail Host: [smtp-server-address]
- Default User: the username to authenticate on the smtp server
- Default Return Address: the address used by recipients to reply the message. Some servers require that this address should be the one used by the authenticated user to access his mailbox.
If the server doesn’t request secure authentication, then the three steps above are enough to start using the new JavaMail session, but a server without secure authentication is a very rare case nowadays. You will certainly need to inform a password to login on the smtp server. In most cases, the server administrator also changes the default port of the smtp server, which forces us to explicitly inform the correct port. For these special needs we can use additional properties in the JavaMail session. Follow the steps below:
- Still on the JavaMail session form, go to the Additional Properties section and add 3 more properties, which are:
- mail.smtp.port: [port-number]
- mail.smtp.auth: true
- mail.smtp.password: **
- Click on Save to create the JavaMail session.
The last step is how to use this new JavaMail session in our applications to send emails. Using the JNDI name, we are going to inject the JavaMail session in a Java class, which could be a POJO of a pure web application, an EJB Session Bean, or any other type of class. See the code below for details:
public class UserAccountBsn {
@Resource(name = "mail/[email-account-name]")
private Session mailSession;
public void sendMessage(UserAccount userAccount) {
Message msg = new MimeMessage(mailSession);
try {
msg.setSubject(“[app] Email Alert”);
msg.setRecipient(RecipientType.TO,
new InternetAddress(userAccount.getEmail(),
userAccount.toString()));
msg.setText(“Hello “+ userAccount.getName());
Transport.send(msg);
} catch(MessagingException me) {
// manage exception
} catch(UnsupportedEncodingException uee) {
// manage exception
}
}
}
The @Resource annotation receives the JNDI name of the JavaMail session and injects an instance of the session in the variable mailSession. This variable is used within the sendMessage method to create a new MimeMessage. The content of the message is built and finally sent to the recipient by the method Transport.send. The method receives as parameter an entity class representing an user registered on the application. It is so simple, isn’t it? 😉
Using this feature, we avoid any additional implementation to add those parameters hardcoded or parameterized, saving a lot of time, simplifying the maintenance of the applications, and reusing existing resources naturally shared by the container.
Recent Posts
Can We Trust Marathon Pacers?
Introducing LibRunner
Clojure Books in the Toronto Public Library
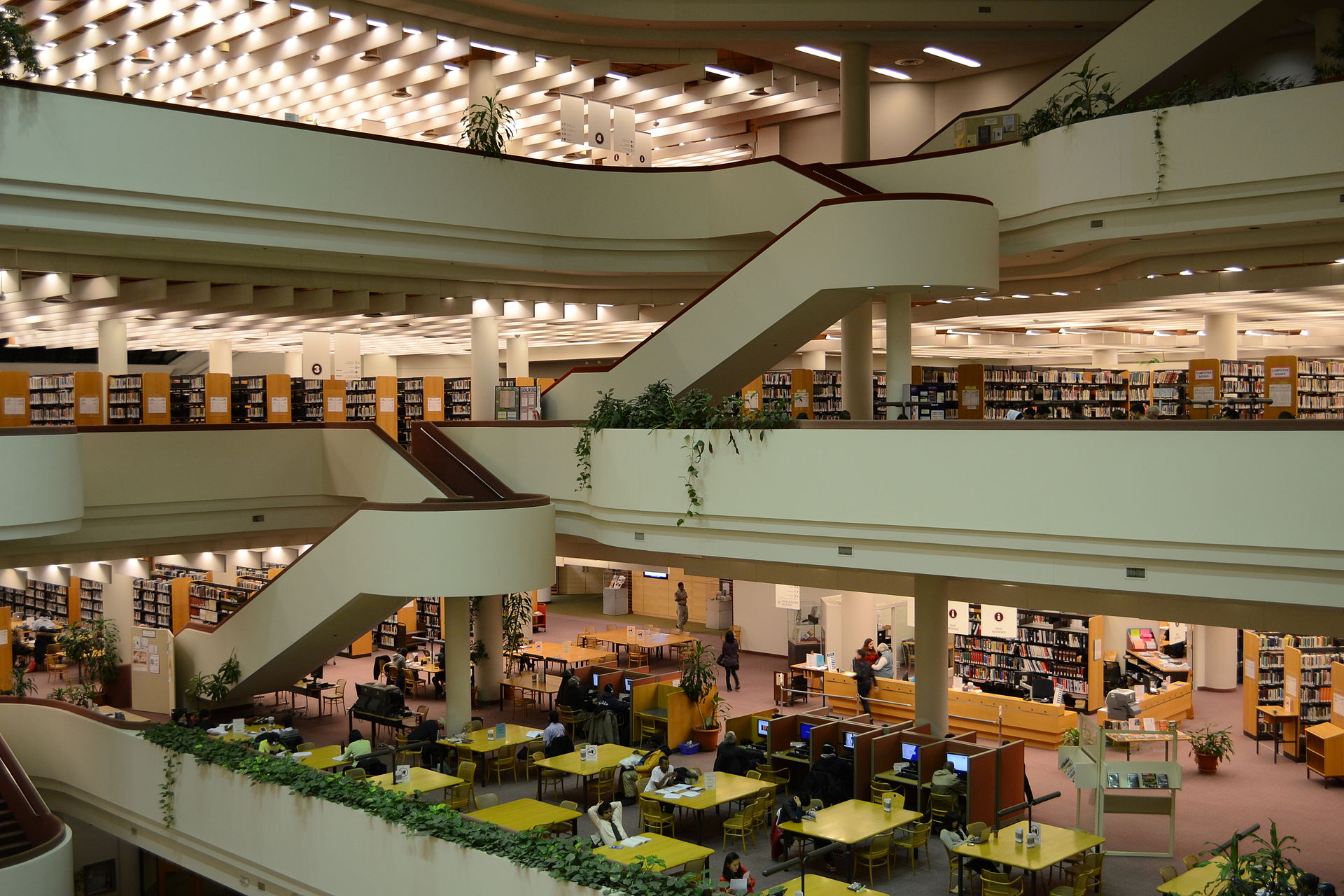
Once Upon a Time in Russia
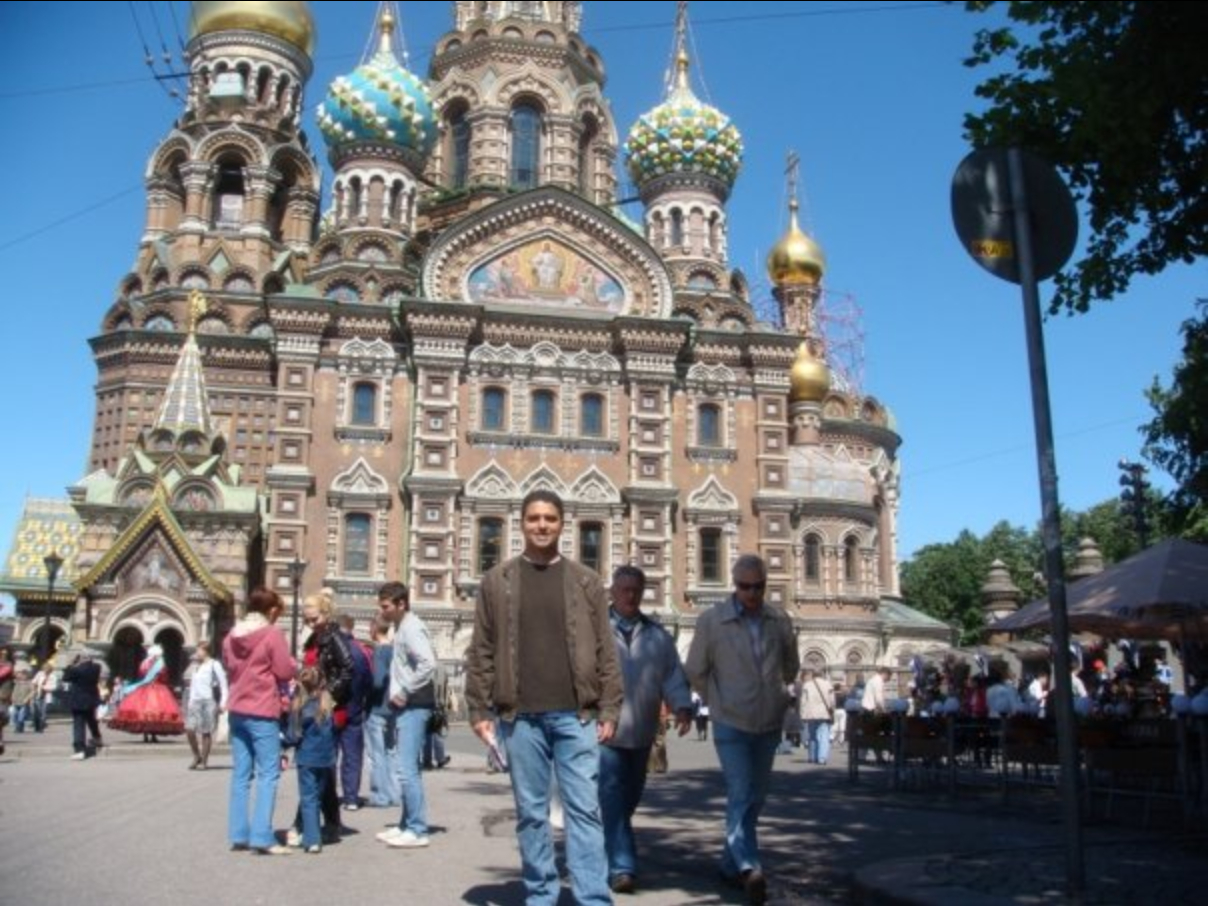
FHIR: A Standard For Healthcare Data Interoperability
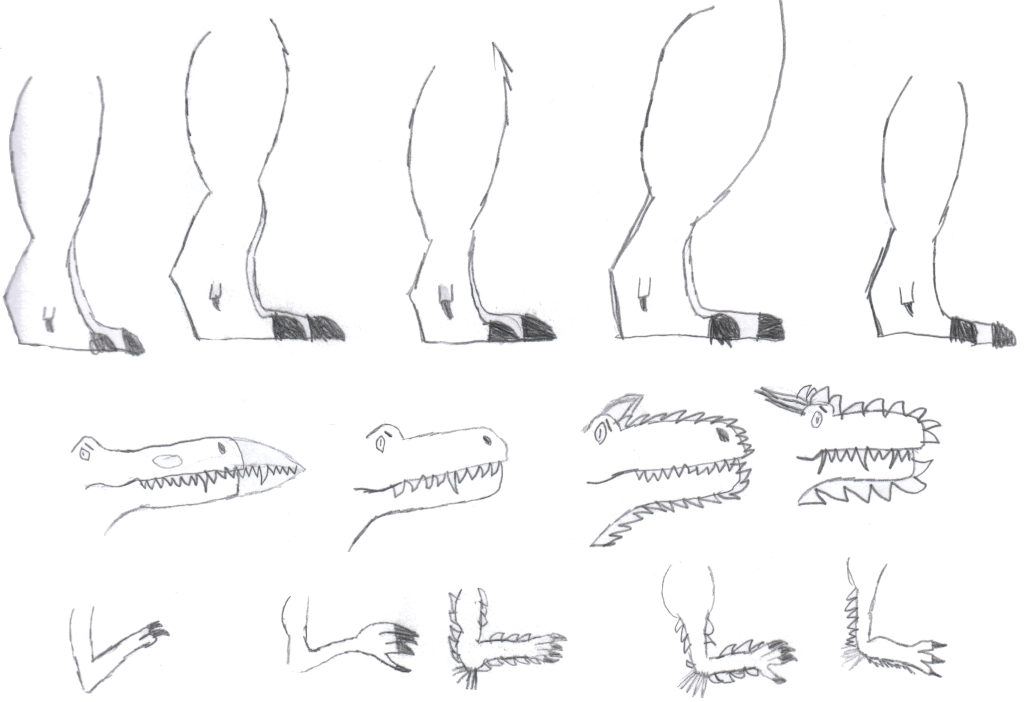
First Release of CSVSource
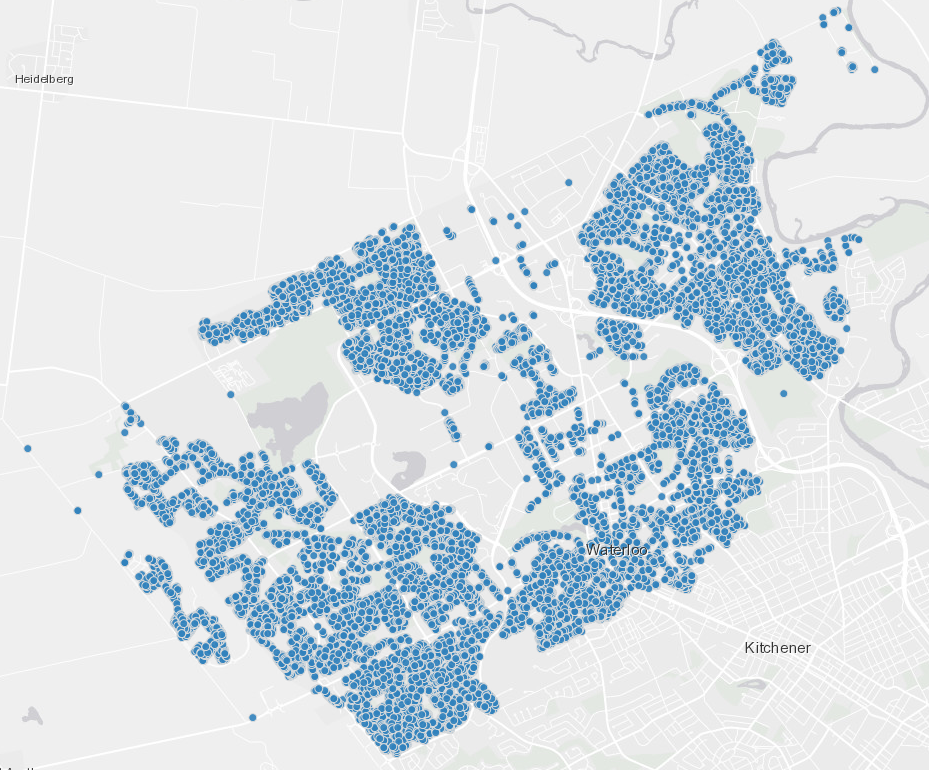
Astonishing Carl Sagan's Predictions Published in 1995
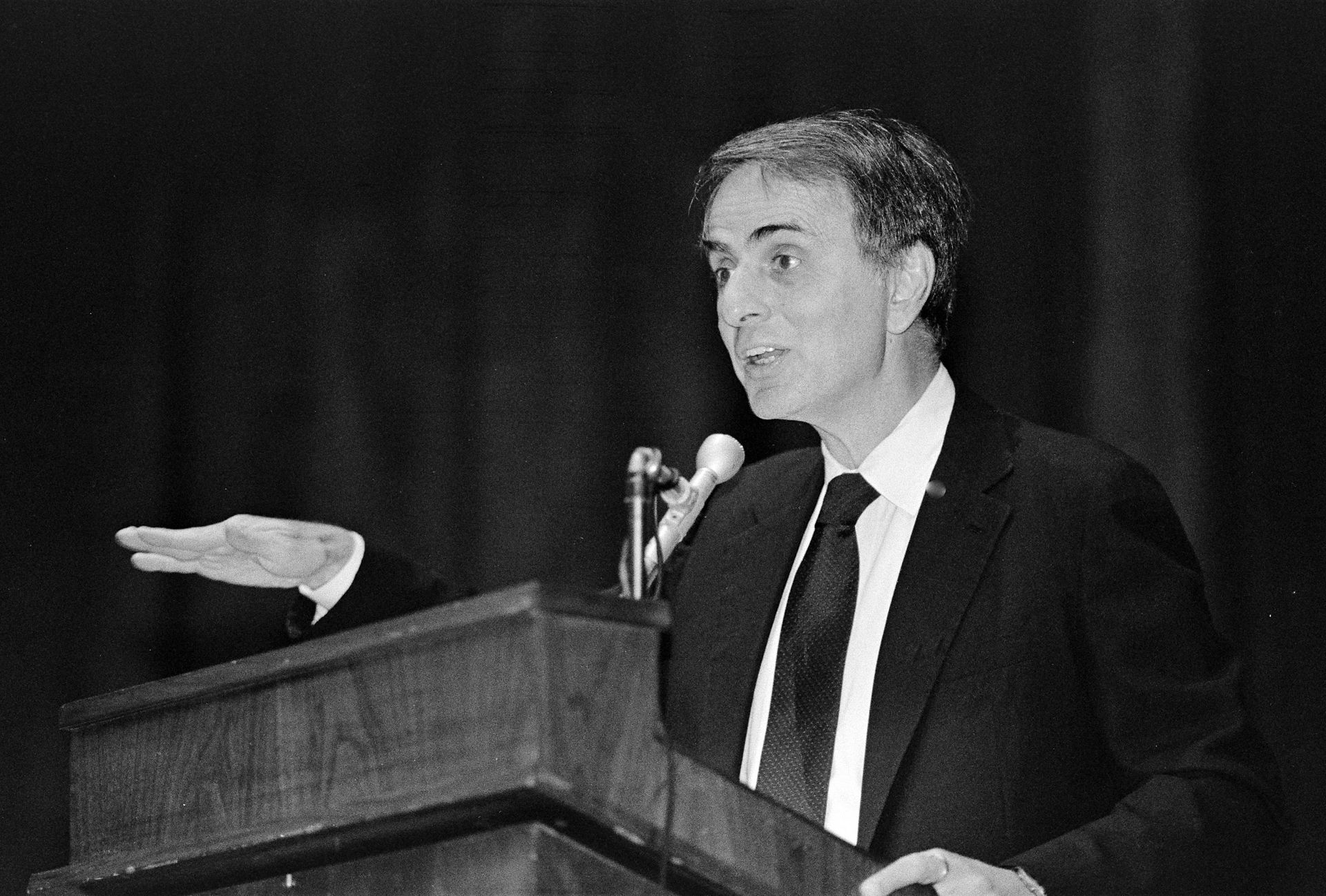
Making a Configurable Go App
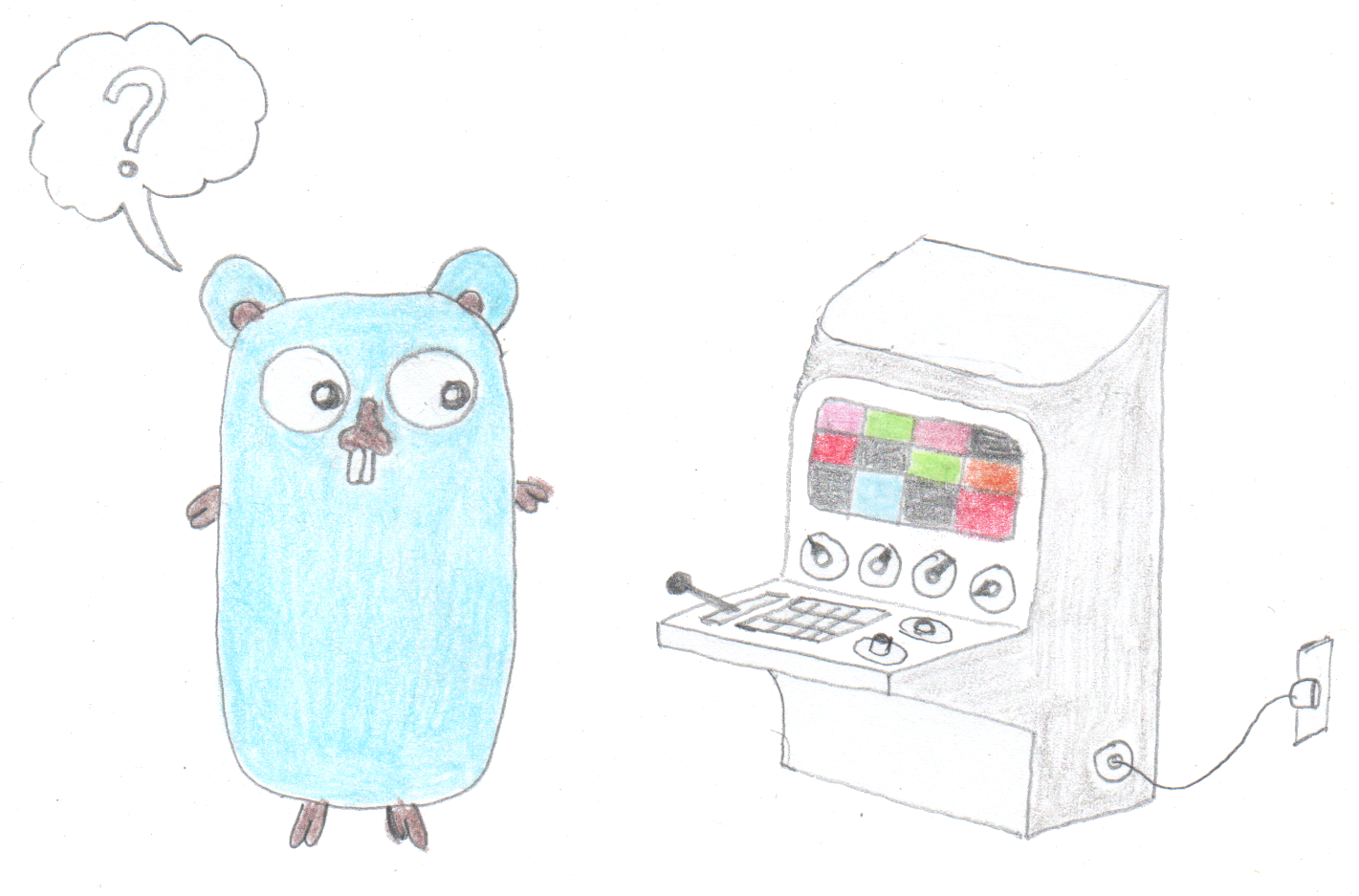
Dealing With Pressure Outside of the Workplace
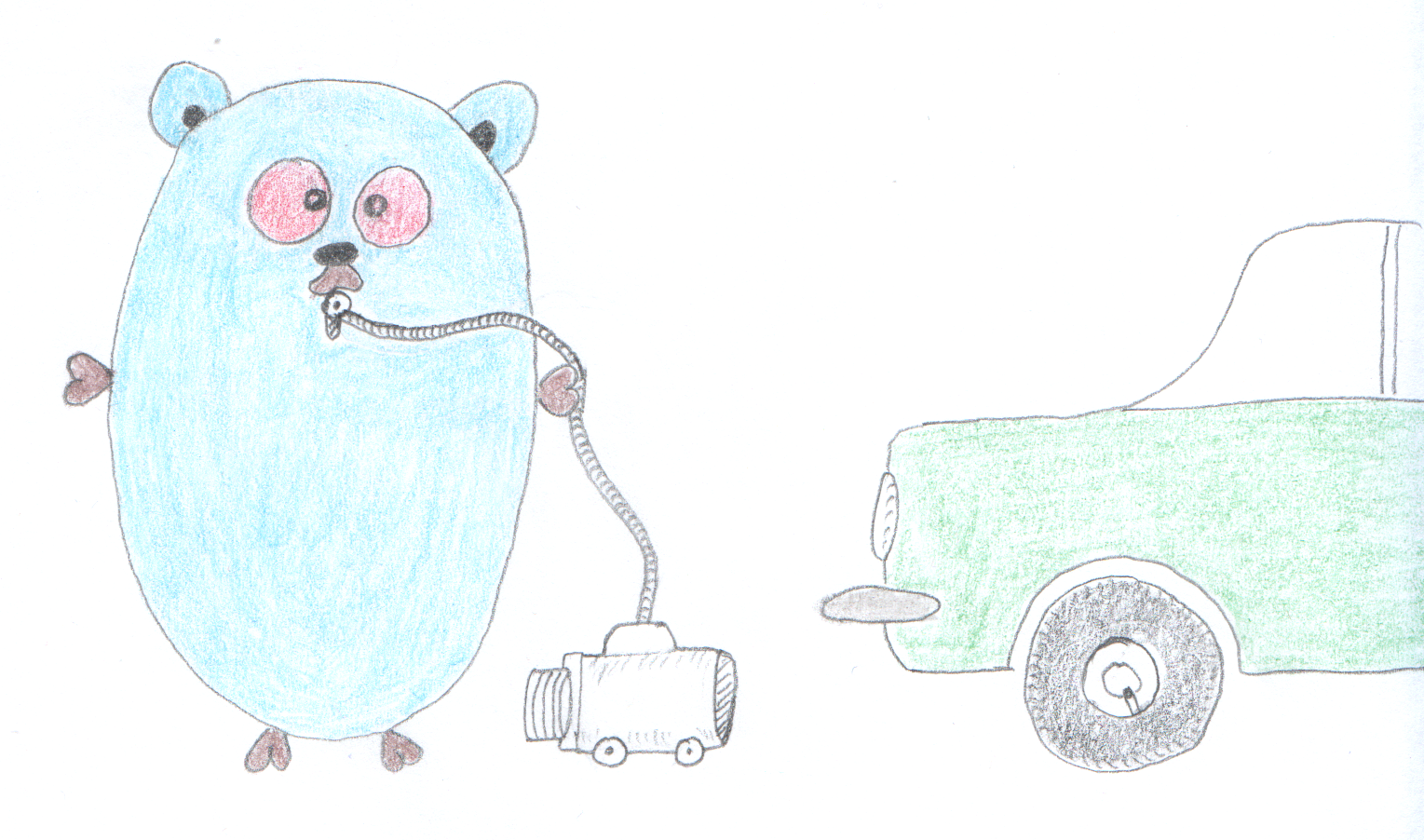
Reacting to File Changes Using the Observer Design Pattern in Go
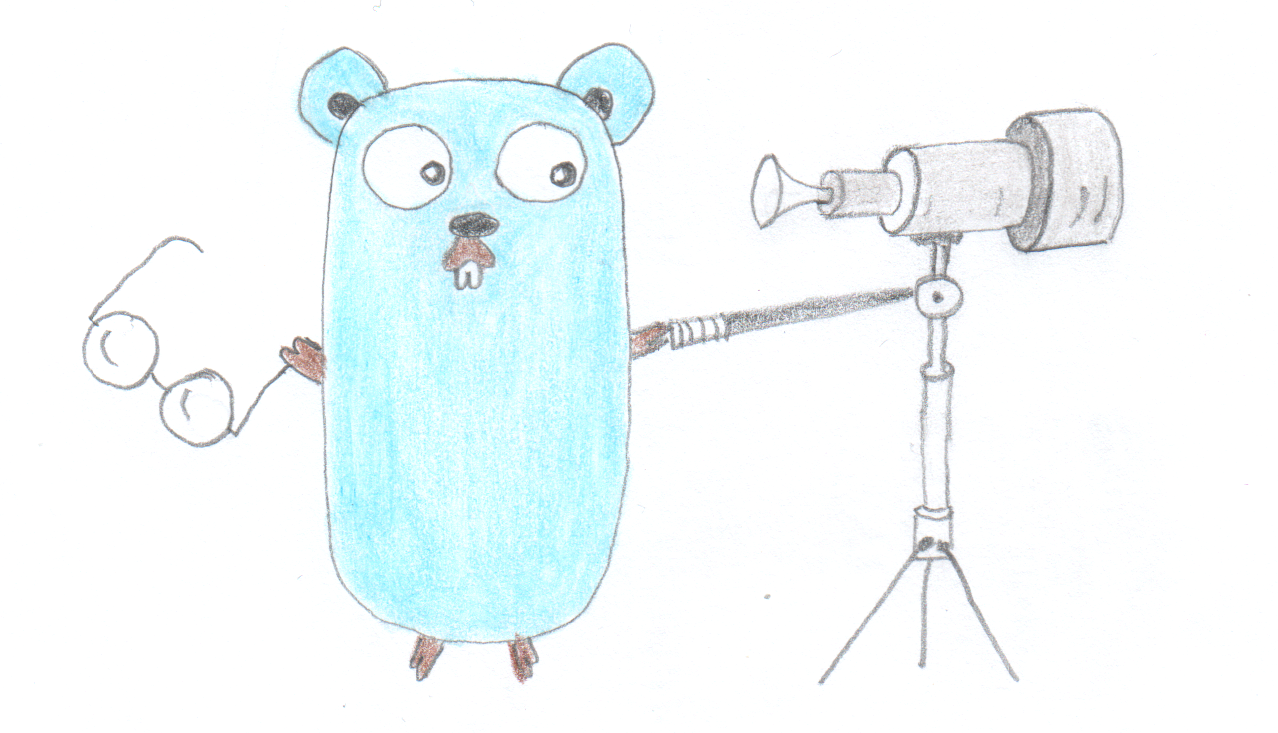
Provisioning Azure Functions Using Terraform
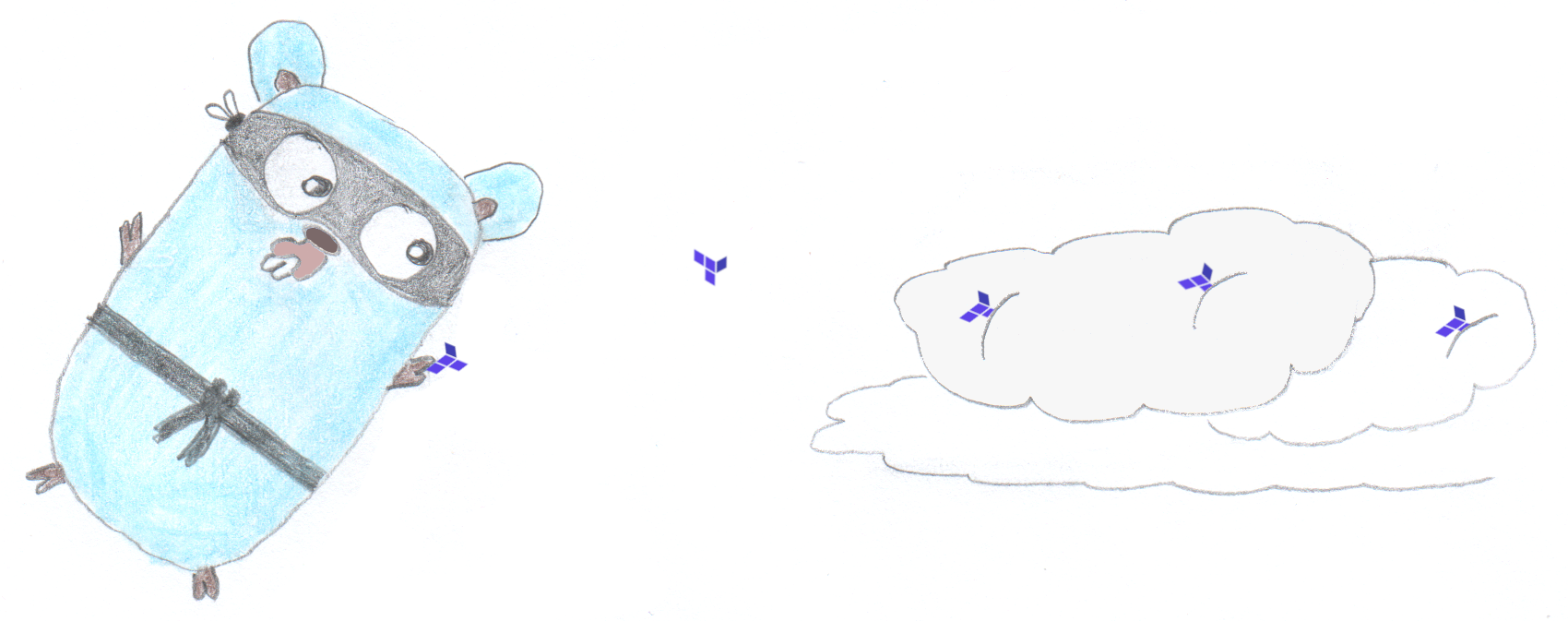
Taking Advantage of the Adapter Design Pattern
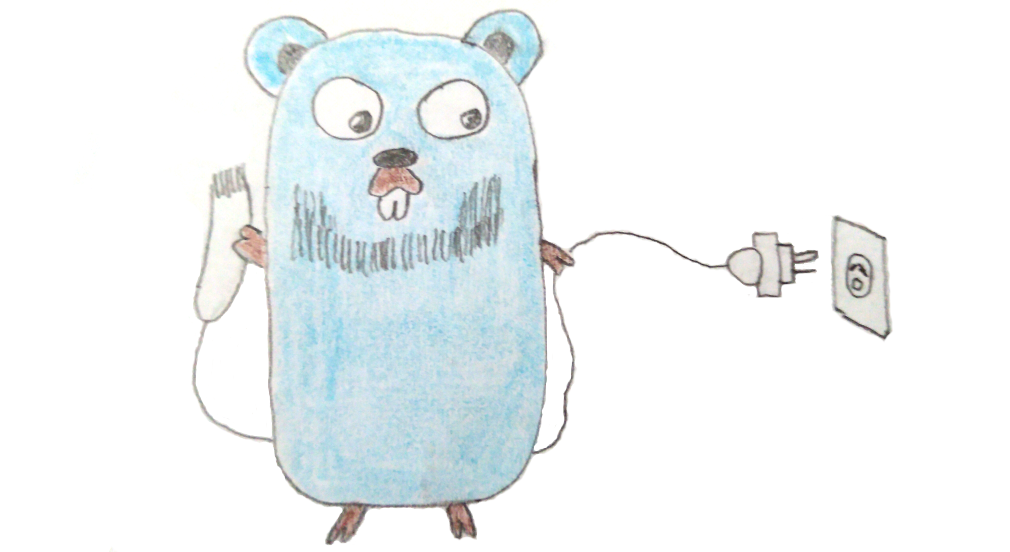
Applying The Adapter Design Pattern To Decouple Libraries From Go Apps
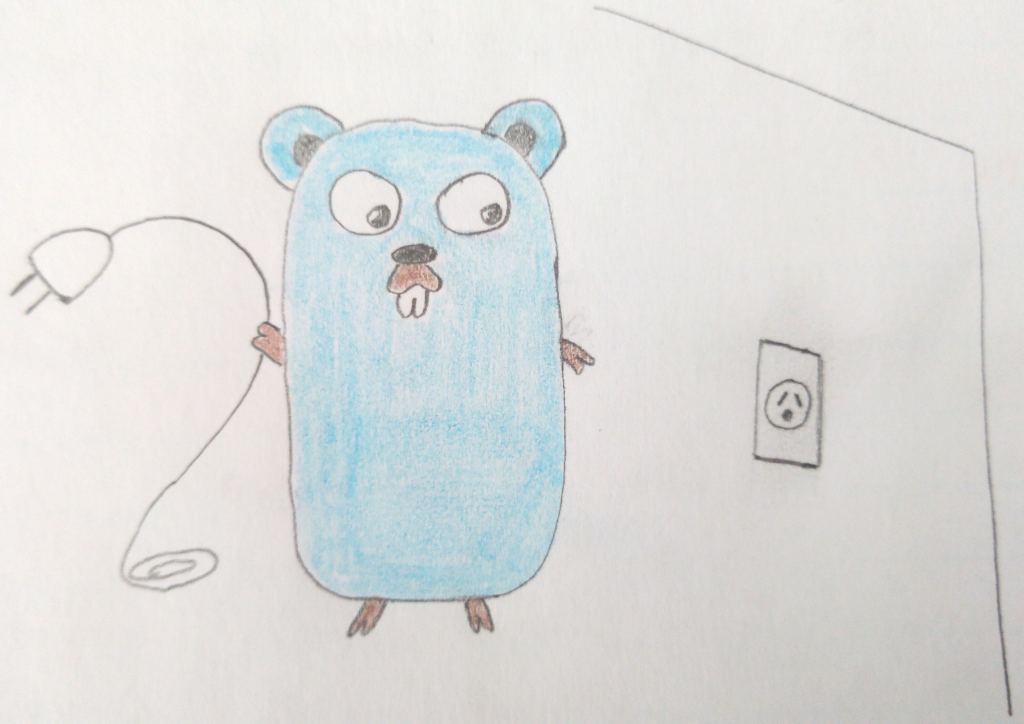
Using Goroutines to Search Prices in Parallel
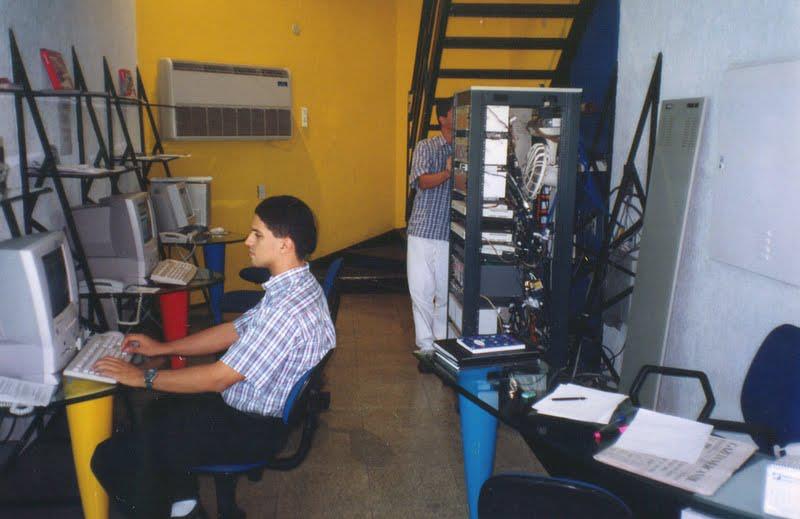
Applying the Strategy Pattern to Get Prices from Different Sources in Go
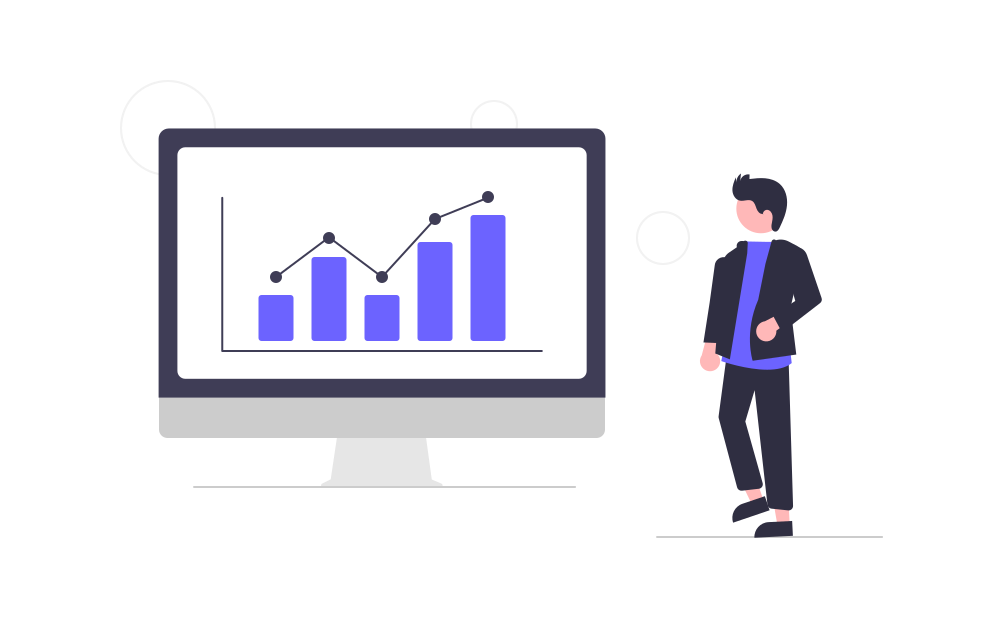